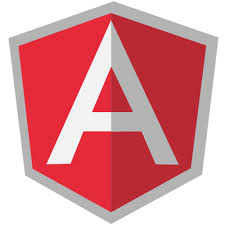
Angular is one of the popular front-end application development platform for building mobile and desktop web
applications. This in-depth Angular training course is specifically designed to meet the day to day development
challenges of a typical Angular Front End Developer.
Course Content
Duration : 32 Hrs
Fees in ₹ : 24000
- Introduction & Getting Started
- What is Angular?
- Angular Development Environment Setup
- Creating the First Project
- What Is TypeScript?
- Understanding The Basics
- Anatomy of an Angular Application
- Angular Components
- Creating the First Component
- Data Binding
- String Interpolation
- Property Binding
- Event Binding
- Directives
- Ternary, Logical, Elvis Operator
- Debugging & Troubleshooting
- Deciphering Error Messages
- Debugging With Chrome Developer Tools
- Inspect Angular App With Augury
- Directives
- Introduction To Directives
- ngFor
- ngIf
- ngStyle
- ngSwitch
- Attribute Directives
- Event Directives
- Structural Directives
- Components
- Introduction To Components
- Creating Custom Components
- @Input & @Output
- Custom Events & Custom Properties
- @ViewChild
- @ContentChild
- Observables
- Introduction To Angular Observables
- Creating Custom Observables
- Styling & Integrating UI Framework
- Integrating Custom Style Sheets
- Introduction To Angular Material
- Working With Angular Material Components
- Advanced Data Binding With Rest API (HTTP)
- What Is a HTTP Request?
- Setting Up a backend with Strapi
- Performing CRUD Operation
- Service With HTTP
- Data Transformation With RxJs
- Error Handling
- Showing A Loading Indicator
- Headers & Query Params
- HTTP Interceptors
- Services & Dependency Injection
- Why Services?
- Creating A Custom Service
- Sharing Data Between Components
- Injecting Service Inside A Service
- Routing & Route Protection
- Angular Navigation & Router Links
- Routes & Parameters
- Default Route
- Custom 404 Page Not Found
- Passing Static Data To Next Page
- Load The Page Based On Data From Backed With Resolve Guard
- Authentication – An Introduction To JWT
- Route Guards
- Nested Routes
- Location Strategy
- Angular Forms
- Creating A Form
- Input Validation
- Reactive Form
- Nested Form Groups
- Form Builder
- Dynamic Controls Using Form Arrays
- Unit Testing
- Why Unit Testing?
- Creating A Unit Test
- Running A Unit Test
- Build & Deployment
- Bundling & Minification
- Tree Shaking
- Lazy Loading
- AOT Compilation
- Deploying The Application
- State Management With NgRx
- Why NgRx?
- Reducers
- Actions
- Effects
- NgRx Dev Tools
- Bonus : Advanced Angular
- Animation
- Offline Capability
- Desktop Apps
- Server Side Rendering
- Pro Tip : Things To Consider On A Large Angular Application